Create CloudFunction
Explanation on how to create an Azure Cloud Function to relay the Venly API calls.
Azure Cloud Functions can be used in combination with PlayFab to manage Server Authoritative calls, so in this guide, we will explain how to create an Azure Cloud Function to relay the Venly API calls. We will walk you through the different install and configuration steps.
There are multiple ways to create an Azure Cloud Function, the explanation below is using Visual Studio (but other IDEs or flow can be used and should be very similar).
Make sure to use our C# PlayFab-Azure Companion Nuget package to set up your Azure Cloud Function. This package will automatically handle the communication with Venly Services and provide access to the full Venly API functions.
1. Create a new Azure Cloud Function
The resulting function should be a HttpTrigger function (.NET6), with the 'Function' authorization level.

New Azure Cloud Function
2. Default Azure Function
Create a new default Azure Function, and remove any template code inside this function if needed. Make sure to give your function a decent name, this name should be defined with the FunctionName
attribute. In this case, our Function is called Execute
.
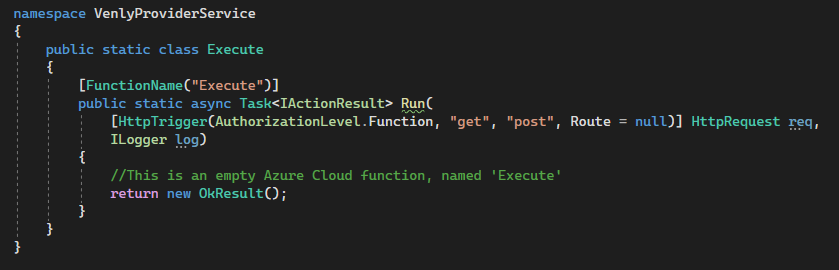
A new default function called Execute
3. Install the Venly Companion assembly
If you haven't done so yet, install the Venly Companion package for PlayFab-Azure. This package will automatically handle the communication with Venly Services and provide access to the full Venly API functions. The easiest way to set it up is by using our VenlyAPI.Companion.PlayFab-Azure Nuget package. Install the Nuget package into your project.
dotnet add package VenlyAPI.Companion.PlayFab-Azure
4. Update the Azure Function body
Now we can replace the initial microservice template code with our logic. This means,
- Calling the appropriate functions to handle our incoming requests.
- Optional, configuring the Endpoint Guard (system that allows you to configure access to certain endpoints)
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Logging;
using Venly;
using Venly.Companion;
namespace VenlyProviderService
{
public static class Execute
{
[FunctionName("Execute")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
//(optional) Here you can also configure the Endpoint Guard
//Changing the default behavior of what endpoints are allowed/denied
VenlyAPI.Backend.AllowEndpoint(VenlyAPI.Wallet.IDs.ExecuteCryptoTokenTransfer);
VenlyAPI.Backend.AllowEndpoint(VenlyAPI.Wallet.IDs.ExecuteMultiTokenTransfer);
VenlyAPI.Backend.AllowEndpoint(VenlyAPI.Wallet.IDs.ExecuteNativeTokenTransfer);
//...
return await VenlyAzure.HandleRequest(req);
}
}
}
And that is all! You can now Publish your function to the Azure Cloud! After publishing we still need to provide our function with some authentication information such as our Client Credentials, and PlayFab Developer Keys.
5. Azure Cloud Function Configuration
Head over to the Azure Portal, and select your Azure Function App from the portal. Here you should be able to configure some Key-Value pairs (Settings > Configuration).
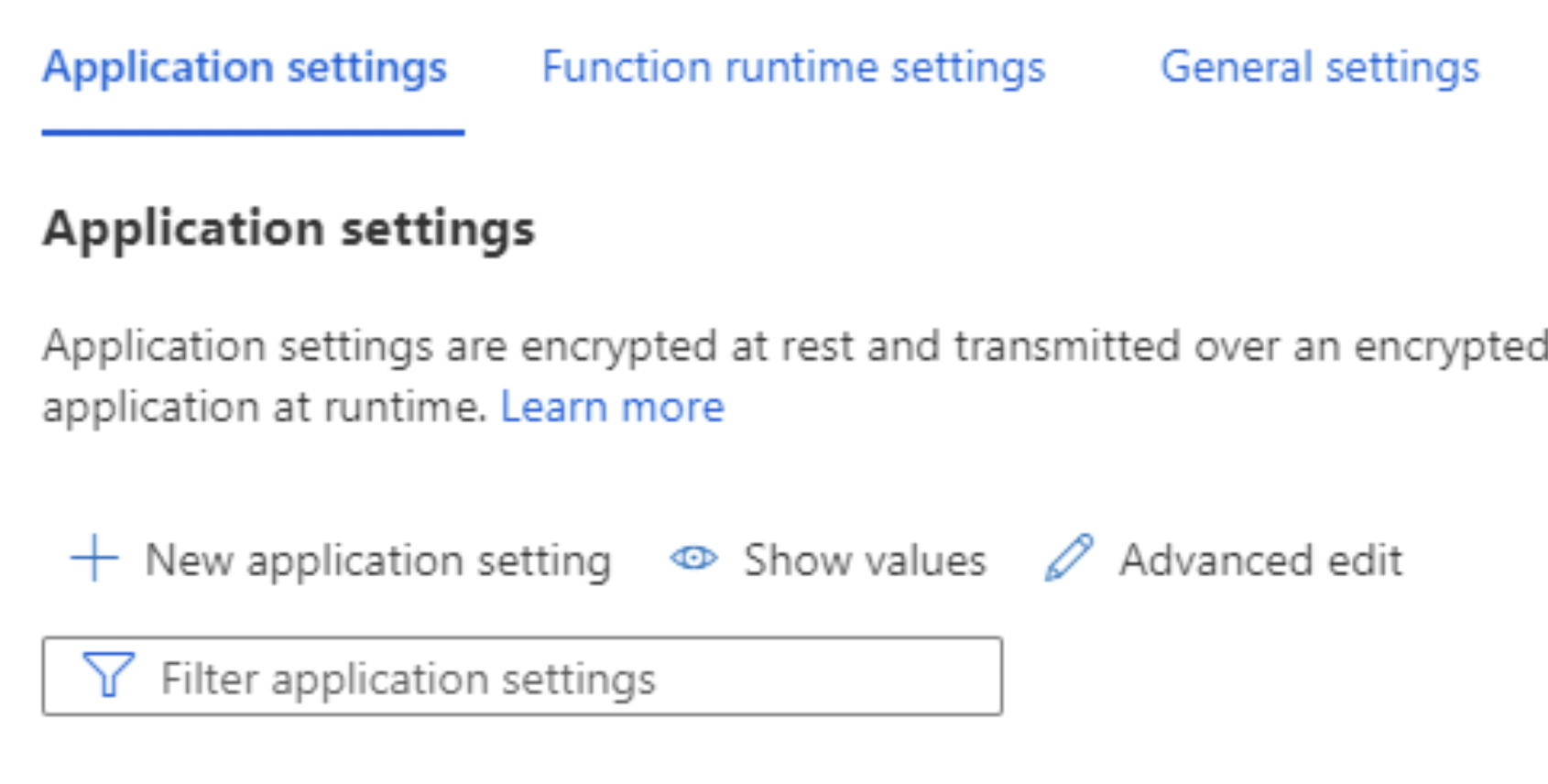
Settings > Configuration menu
Here we have to create a few additional settings that will provide our Function app with some required information to function properly. The following keys should be added:
Make sure to use the exact same name for the keys!
KEY | VALUE |
---|---|
VENLY_CLIENT_ID | Your Venly Client ID |
VENLY_CLIENT_SECRET_STAGING | Your Staging Client Secret |
VENLY_CLIENT_SECRET_PRODUCTION | Your Production Client Secret |
VENLY_ENVIRONMENT | Either STAGING or PRODUCTION |
PLAYFAB_TITLE_ID | Your PlayFab Title ID |
PLAYFAB_DEV_SECRET_KEY | Your PlayFab Developer Secret Key |
During development it is sufficient to only provide the Staging Client Secret - this means you also have to make sure that the Environment is set to
STAGING
.
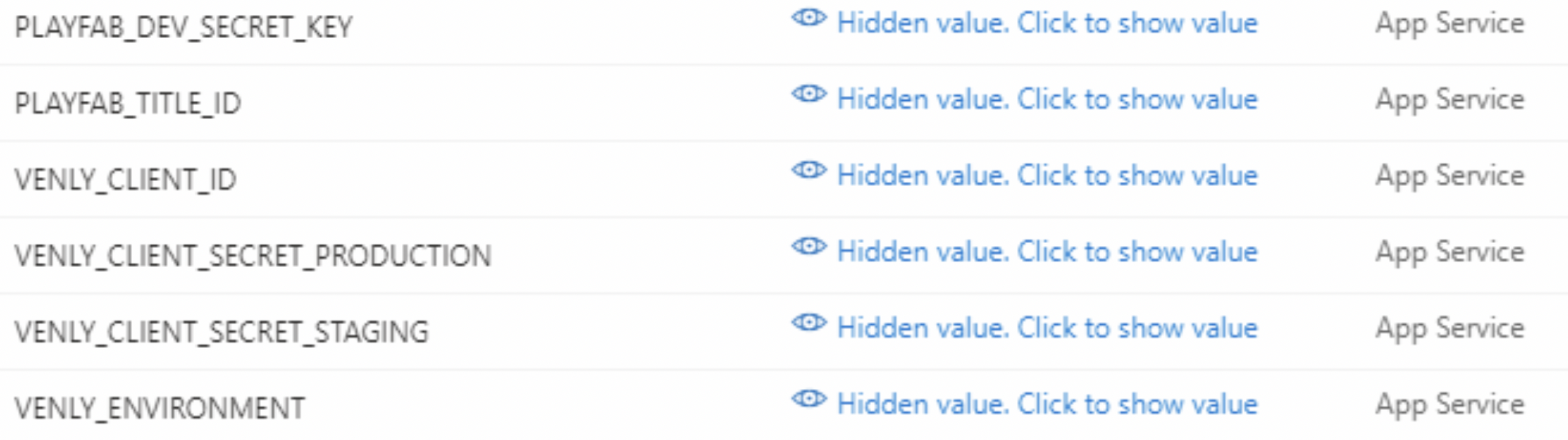
Azure Function - Application Settings
Updated 8 months ago