Build an in-app NFT market
The Market-API provides all the tools needed to create an in-app marketplace. This page describes the needed steps and parts.
Context
The Venly Market-API takes care of the in-custody / buying/selling process of NFTs. From a high-level perspective, the only thing that your app needs to do is:
- Supply users and their balance
- Create offers
- List offers
- Buy offers
Before you start
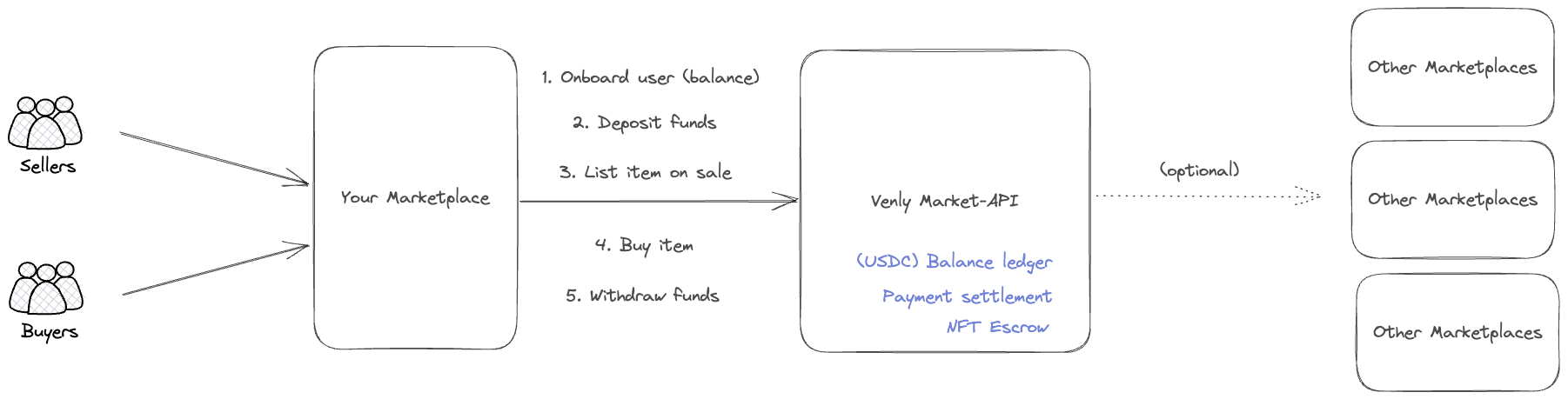
Venly Market Flow
The Venly Market is a custodial marketplace that supports buying and selling of NFTs with USDC.
Custodial Marketplace
This means that we take an NFT into escrow whenever it is put on sale. This ensures that the NFT will actually be available for purchase, during the lifespan of the offer. Once an offer is bought, the NFT will be handed over from our escrow wallet to the buyers-wallet. A seller can at any time cancel an offer. As a result, the NFT will be sent back to the sellers-wallet.
Buying and Selling NFTs
The basic operations of a marketplace are buying and selling. We support regular sales, as well as auctions. NFTs are put on sale by the owner of the NFT. Any seller can hereafter purchase the offer. When a sale is fulfilled, the buyer will receive the NFT, the USDC balance of the buyer will be diminished with the sales price and the balance of the seller will be increased with the sales price (minus fees).
USDC
Our marketplace works with USDC. USDC is a stablecoin, linked to a US-Dollar value. The balance of an end user, the offer price, and bids on auctions are all done in the USDC currency (independent of the blockchain of the NFT).
This means that, for a user to be able to buy an NFT, they need to have a positive USDC balance in the marketplace. Users can deposit and withdraw USDC in their market account.
When an NFT is bought or sold, the USDC balance of the buyer will be diminished with the offer price, and the USDC balance of the seller is increased with the offer price (minus fees).
Overview
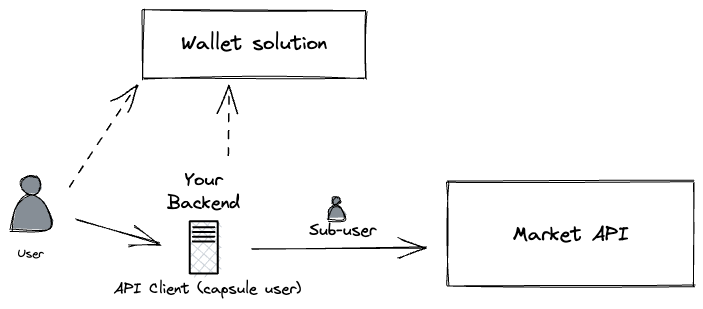
User Flow for Market API
To interact with the Market-API (buying and selling items), you will need a Wallet solution. This is because you will need to sign a blockchain transaction when creating offers, and bought NFTs need to be transmitted to a buyer's wallet.
The Market-API is made Wallet-agnostic. This means that you can use any wallet solution you prefer. Of course, we recommend Venly Wallet solutions (API or Widget), but you are free to choose any other wallet provider (e.g. Metamask). The only requirement is that the chosen solution is able to sign raw data, EIP712 (meta-transaction) messages and execute blockchain transactions.
Every action done on the Market-API is done with your Client ID and Client Secret. This means that the Market-API, in its purest form, does not know your end users. It is your app that creates the offers and buys them.
Of course, in most cases, you provide a marketplace for your in-app end users. It is those end users that are buying and selling. Therefore, a translation must be made between your clientID
and the actual end-user. This can be done with the Sub-Users concept.
Flow
We'll outline the steps you need to take to create a fully operational in-app marketplace.
Onboarding users
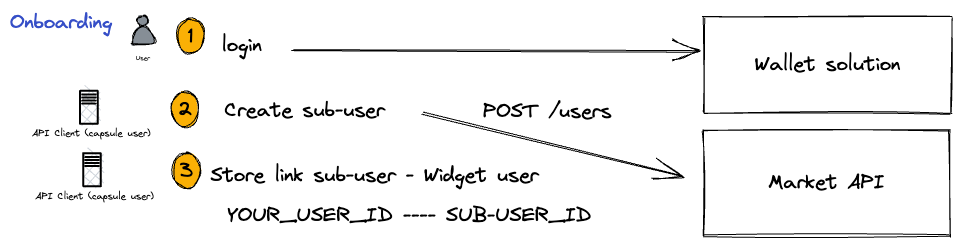
Onboarding Sub-Users Flow
Each user in your system can be a seller or buyer in your in-app marketplace. Each user, therefore, needs to have its own market balance (USDC).
In order to achieve this, you will need to create a sub-user for each of your users. Each sub-user will receive its own market balance that can be used for buying and selling.
Create offers

Create Offers Flow
When a user is onboarded as a sub-user, they can place NFTs on sale (create offers). You can add the sub-user details of the seller when creating an offer.
See the offer creation flow to know how to sign for the offer so that Venly can take the NFT into escrow. Note that, when working with sub-users, it is the actual owner of the NFT that needs to sign for the in-escrow transaction. You will therefore need to let the actual end user sign this transaction with the wallet solution you have integrated.
Topping up the sub-users market balance

Topping Sub-User's Balance
Before a buyer can buy an item, the buyer needs to have a positive USDC market balance. For this, the user (or you) can deposit USDC in a specific deposit address assigned to the sub-user. The deposit address can be retrieved or created with specific endpoints.
Use your chosen wallet solution to transfer USDC to the user's deposit address.
Buying items

Buying Items on Market
When the buyer has a positive market balance, a Fulfillment can be created, specifically for that sub-user.
Hereafter, the Venly escrow system takes over. The balance of the buyer and seller will be updated and the NFT will be transmitted to the provided walletAddress
.
References
Sub-Users
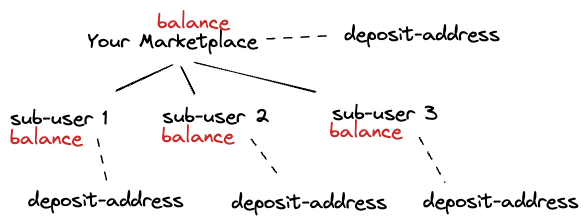
Sub-Users Concept in Market
With your clientId, you can create sub-users
on the Market. Sub-Users are handled the same way as regular users: they have a market balance (USDC), deposit USDC, withdraw USDC, buy items, and sell items.
The only difference is that sub-users
do not have an active Venly-account. You are in charge of identifying and authenticating your end users. For Venly, a sub-user
is merely a technical concept and all API calls originate from your clientID
.
So, depending on your use case, you can create sub-users in Venly. You can also choose to handle everything on your side (in that case you will need to keep a balance ledger on your side).
Sub-user creation
Sub-users can be created with the endpoint:
Request Endpoint: reference
POST /users
Request Body:
{
"type": "SUB_USER",
"nickname": "string"
}
After creation, you will have to link the acquired sub-user-id to the user-id in your own system. This way, you can correlate your users with sub-users in the Market-API and make the correct subsequent calls (selling and buying items).
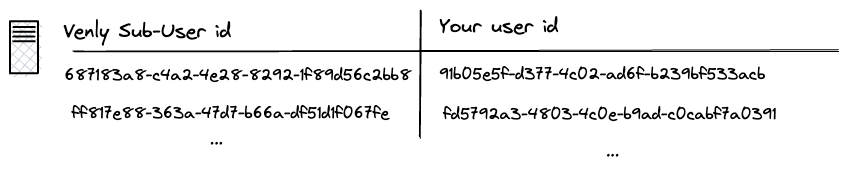
Link Sub-User id
Sub-users balance
Besides your main balance, each sub-user gets a separate market balance (in USDC). This balance will be used when you initiate buying or selling for your sub-users.
This means that, before a sub-user can buy an offer, that sub-user needs to have a positive market balance. Check a sub-users balance by:
Request Endpoint: reference
GET /users/{userId}/balance
USDC can be deposited in the market balance of the sub-user by transferring USDC to the specific deposit address of the sub-user. To create a deposit address for your sub-user, call the endpoint:
Request Endpoint: reference
POST /users/{userId}/deposit-addresses
Showing the available offers
You can view all the offers that you have bought or sold and filter the responses, using the following endpoint.
Request Endpoint: reference
GET /user/offers
Closed or Open Offers
An important decision you have to make when creating an in-app marketplace is if you want to have your created offers publicly available, or not.
This can be set with the visibility
parameter on an offer.
Basically:
PRIVATE
offers are for you and you alone. These offers are only visible in your in-app marketplace. No one else can see or buy these offers.PUBLIC
offers are searchable, and retrievable in other marketplaces that implement the Venly Market API. Our own Venly Marketplace is such a case. When you create aPUBLIC
offer through your in-app marketplace, that same offer will also show up in the public-Venly Market.
Updated 5 months ago