User Management
This guide will take you through the API calls for creating, deleting, updating, and managing users.
⚠️Disclaimer!
All wallets created will be non-custodial.
When you create a user and link a wallet to them, we recommend creating an additional signing method (EMERGENCY CODE). This ensures that your users have a means to reset their PIN in case it is lost.
Failure to create an emergency code means that if a user loses their PIN, they will permanently lose access to their wallet.
Context
Throughout this guide, when we mention "Users," we are referring to the individuals who will be using your service as end-users.
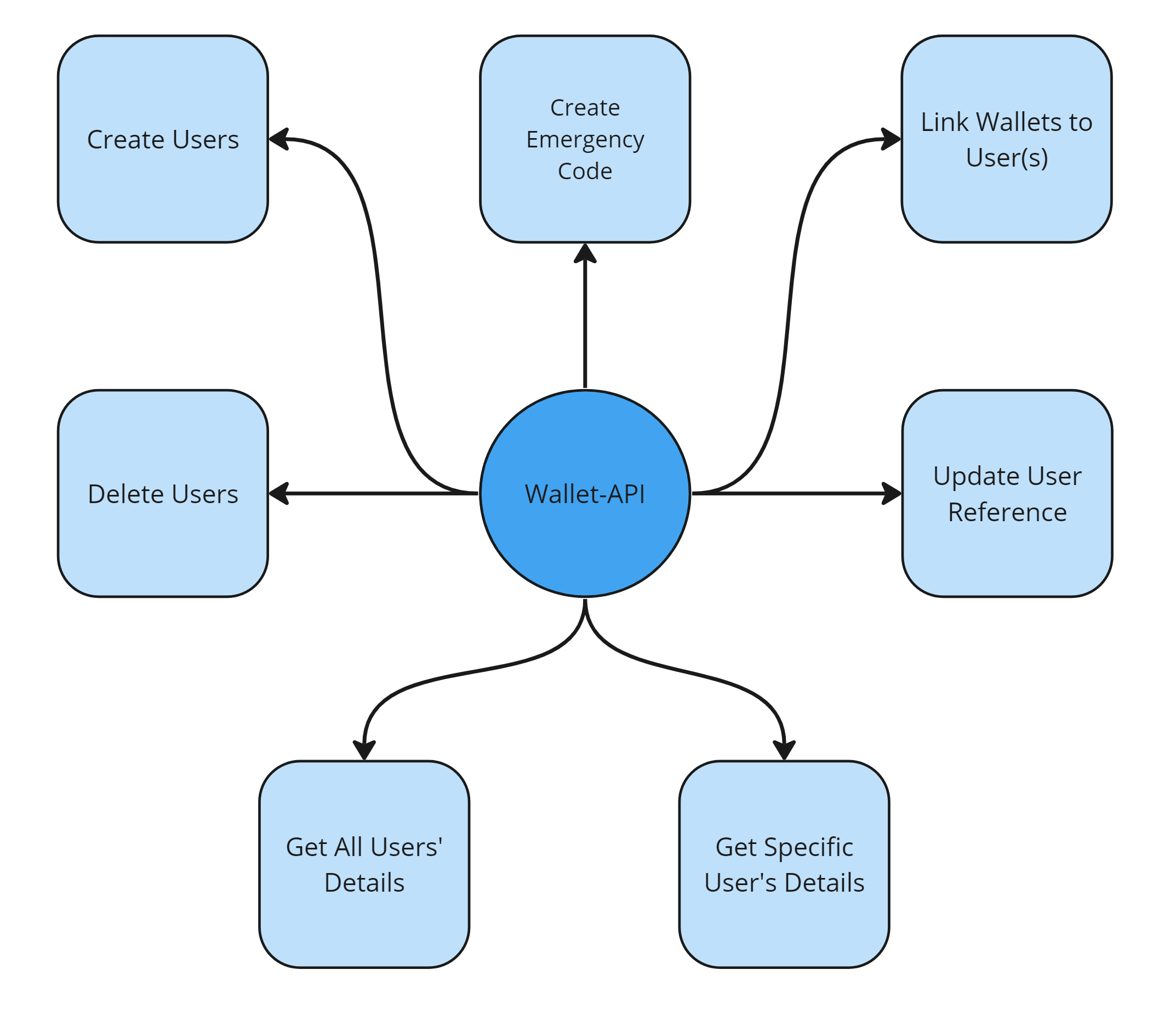
User Management - Wallet-API
Introduction
Each User corresponds to one of your end-user. If you don’t have end users, you can still use the “User” concept without those corresponding to actual end users.
You can create multiple Users, and all of them can have a different signing methods and multiple wallets linked to them via Wallet-API.
You can create:
- One User and have multiple wallets linked to them
- Multiple users and all of them can have one or more wallets
The Wallet-API allows you add a reference
when creating a User. This reference
parameter can be virtually anything that describes a User. It helps you tell one User apart from another.
Let's see how you can create a User.
1. Create a User
You can create one or multiple users using the same flow. If you create only one user and link all wallets to it, then all wallets will be accessible with the signing methods you create for this user.
The
signingMethod
object in body is optional. You can create users without a specifying a signing method.
For this example, we will create a signing method along with creating a user.
To create a user, call the following endpoint:
Request Endpoint: reference
POST /api/users
Request Body:
Parameter | Type | Required | Description |
---|---|---|---|
reference | string | ❌ | You can provide a reference (can be whatever you want) to distinguish between users. |
signingMethod | object | ❌ | This object contains the type of signing method and it’s value. |
type | string | ❌ | This parameter indicates the type of signing method. (e.g., PIN, EMERGENCY_CODE, BIOMETRIC.) The first signing method should always be a PIN. |
value | string | ❌ | This is the value of your signing method. For example, the value for PIN can be “123456”. |
{
"reference": "ABD User 1",
"signingMethod": {
"type": "PIN",
"value": "123456"
}
}
Response Body:
Save the following parameters from the response body. They will be used later in the guide.
- result.
id
: This is the User ID. Every user you create will have a unique user ID. - signingMethods.
id
: This is the signing method ID and will be used for authentication purposes. Every signing method will have a different and unique ID.
It is IMPORTANT to save the PIN signing method ID since it is used during the PIN reset process, or when creating an additional signing method.
{
"success": true,
"result": {
"id": "e85470a8-5deb-41f6-be6c-de693683a22c",
"reference": "ABD User 1",
"createdAt": "2023-08-02T07:18:58.624685414",
"signingMethods": [
{
"id": "4658c064-05b9-4bbd-9f0e-68a9588c7bc3",
"type": "PIN",
"incorrectAttempts": 0,
"remainingAttempts": 10,
"hasMasterSecret": true
}
]
}
}
2. Create Emergency Code (recommended)
In this example, we will create an additional emergency code signing method. This emergency code can be used to reset a forgotten PIN code, sign transactions, and to authenticate calls.
When creating a signing method for a user for the first time, you don’t have to provide another one.
When creating additional signing methods, you will have to provide an existing valid signing method in the header.
Call the following endpoint to create an emergency code:
Request Endpoint: reference
POST /api/users/{userId}/signing-methods
Path Parameters:
{userId} : This is the User ID whose signing method you want to create. Paste the previously saved User ID here.
Header Parameters:
For this example, the user already has an existing PIN signing method, that's why we are providing the PIN signing method in the header request to authenticate the call.
Parameter | Value | Description |
---|---|---|
Signing-Method | id:value |
|
Request Body:
{
"type": "EMERGENCY_CODE"
}
The body does not contain the value
property because we want to autogenerate the emergency code.
Parameter | Type | Description |
---|---|---|
type | string | This parameter indicates the type of signing method. (e.g., PIN, EMERGENCY_CODE, BIOMETRIC.) |
value | string | This is the value of your signing method. For example, the value for EMERGENCY_CODE can be “w2v7yertaad21lhudqghzwcg4”. To autogenerate the emergency code, this field can be removed. |
For this example, we are creating the emergency code signing method.
Response Body:
It is IMPORTANT to save the PIN signing method ID since it is used during the PIN reset process.
Description of parameters under the result
object:
- result.
id
: This is the signing method ID - result.
value
: This is the value of the generated emergency code
{
"success": true,
"result": {
"id": "3eccfcef-467a-42a6-8b6b-6ffce3b3b115",
"type": "EMERGENCY_CODE",
"incorrectAttempts": 0,
"remainingAttempts": 10,
"value": "taif7dxjikhtb82zm7u93tn26",
"hasMasterSecret": true
}
}
3. Update reference
of a User
reference
of a UserCall the following endpoint and add the new reference
in the request body:
Request Endpoint: reference
PUT /api/users/{userId}
Path Parameter:
{userId} : This is the ID of the User whose reference
you want to update
Request Body:
{
"reference": "Add the new updated reference here."
}
Response Body:
{
"success": true,
"result": {
"id": "9cf9228e-1f2b-4940-9508-4335064cbc76",
"reference": "Add the new updated reference here.",
"createdAt": "2023-08-07T14:04:54.262619"
}
}
4. Get Details of all your Users
This endpoint can be used for getting all users you’ve created and their signing methods:
Request Endpoint: reference
GET /api/users
Query Parameters:
Parameter | Type | Required | Description |
---|---|---|---|
page | integer | ❌ | This parameter is used to specify the page number. |
size | integer | ❌ | This indicates the number of users to output per page. |
sortOn | string | ❌ | The name of the field that you want the results to be sorted by, such as createdAt . |
sortOrder | string | ❌ | This is to show the results in ascending or descending order. The value for this can only be ASC or DESC . The default value is ASC . |
reference | string | ❌ | This is used to filter your users based on the labels(reference ) you've assigned. |
includeSigningMethods | boolean | ❌ | This "Boolean" indicates whether to display the details of signing methods for users or not. |
Response Body:
{
"success": true,
"pagination": {
"pageNumber": 2,
"pageSize": 1,
"numberOfPages": 10,
"numberOfElements": 10,
"hasNextPage": true,
"hasPreviousPage": false
},
"result": [
{
"id": "00f008b1-5e78-4f3b-9d41-8d11d953497a",
"reference": "d",
"createdAt": "2023-07-28T10:19:19.184984",
"signingMethods": [
{
"id": "dc3218e9-a8e8-4dca-a8cb-66ebfd2701a6",
"type": "EMERGENCY_CODE",
"incorrectAttempts": 0,
"remainingAttempts": 10,
"hasMasterSecret": true
},
{
"id": "ca265520-e33b-4d96-91b7-cc3eceee345d",
"type": "PIN",
"incorrectAttempts": 0,
"remainingAttempts": 10,
"hasMasterSecret": true
}
]
}
]
}
5. Get details of a specific User
This endpoint can be used for getting information about a specific user you’ve created and their signing methods:
Request Endpoint: reference
GET /api/users/{userId}
Path Parameter:
{userId} : This is the ID of the specific User whose information you want
Response Body:
{
"success": true,
"result": {
"id": "9b4414c7-d033-4cba-9635-a535d31d7030",
"reference": "USER-A",
"createdAt": "2023-08-03T14:23:16.037191",
"signingMethods": [
{
"id": "3355c892-31af-4260-87a3-a68175bcfea5",
"type": "PIN",
"incorrectAttempts": 0,
"remainingAttempts": 10,
"lastUsedSuccess": "2023-08-04T12:34:36.752094",
"hasMasterSecret": true
},
{
"id": "38ee7ad2-043d-4c38-9b5b-b962d23e7c8e",
"type": "EMERGENCY_CODE",
"incorrectAttempts": 0,
"remainingAttempts": 10,
"hasMasterSecret": true
}
]
}
}
6. Delete a User
This endpoint can be used for deleting a User. If there are any wallets linked to the user, they will be deleted as well.
Request Endpoint: reference
DELETE /api/users/{userId}
Path Parameter:
{userId} : This is the ID of the specific User you want to delete
Header Parameter:
Provide a valid signing method in the header request to authenticate the call.
Parameter | Value | Description |
---|---|---|
Signing-Method | id:value |
|
You will get a 200 OK response indicating the user is deleted.
7. Signing Methods for Users
Each User has a unique signing method which is used for authentication purposes and for signing transactions. They are the gateway to access their wallets.
All Users have a PIN
signing method but they can also have an additional EMERGENCY_CODE
and BIOMETRIC
signing method.
While managing your Users, you can create, update, recover, and delete their signing methods.
Click here for more information on managing Signing Methods for Users.
8. Link Wallet(s) to Users
There are two ways you can link wallets to your users:
Updated 8 days ago