Binding the Microservice
Now that we have created the Venly Microservice and generated the necessary code for our UE project, we need to bind the correct Microservice Function to the Venly SDK. This can easily be achieved by using the VyBeamableProxy::BindExecuteRequest
function.
Binding our Microservice function to the Venly SDK needs to happen before making any calls to one of the Venly APIs.
There are two options to perform this action, through Blueprints or C++.
Using Blueprints
Somewhere at the beginning of your application, add the following nodes to 'relay' all the Venly calls to the corresponding Beamable Microservice. (A separate Blueprint Actor can be used for this)
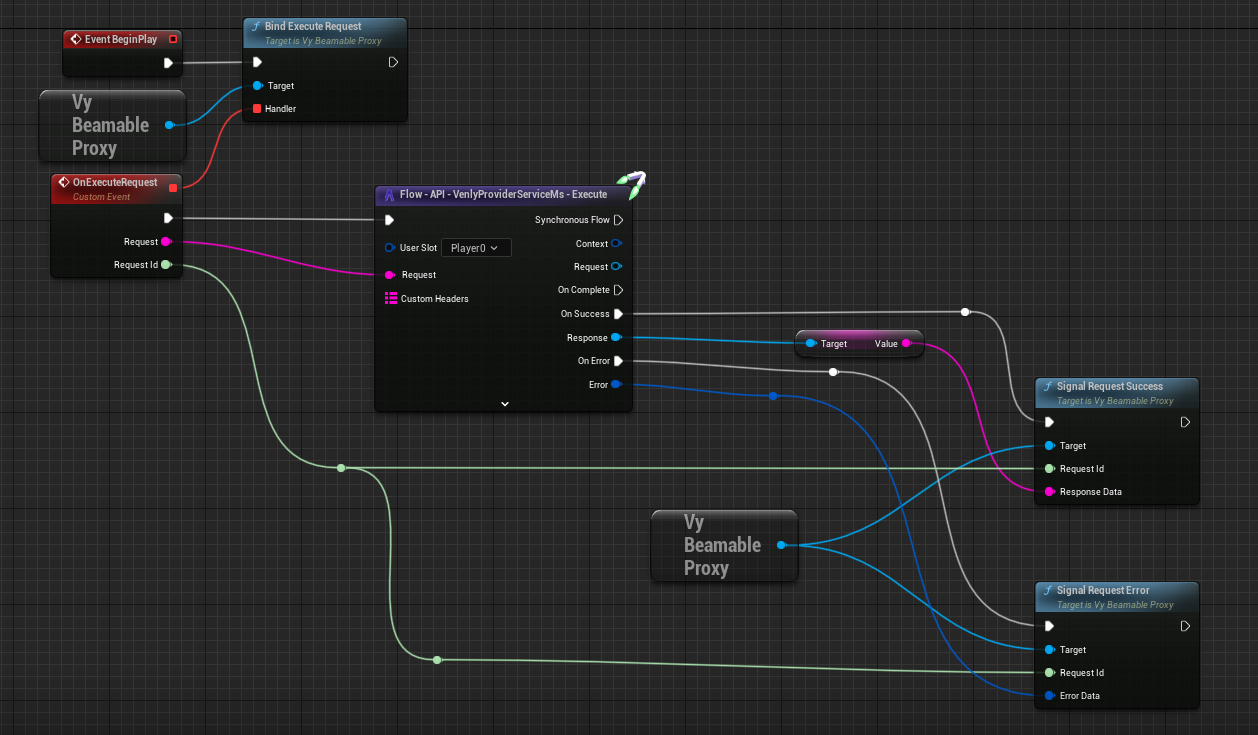
Binding Microservice function to Unreal SDK using Blueprints
Using C++
As an example, we created a new C++ Actor called CPP_BindBeamableMicroservice
. A good spot to bind the Beamable Microservice to the SDK is inside the AActor::BeginPlay
function. The following snippet shows us the binding flow.
#include "CPP_BindBeamableMicroservice.h"
#include "Utils/VyUtils.h"
#include "VyBeamableProxy.h"
#include "AutoGen/SubSystems/BeamVenlyProviderServiceMsApi.h"
//...
// Called when the game starts or when spawned
void ACPP_BindBeamableMicroservice::BeginPlay()
{
Super::BeginPlay();
//Bind the Microservice to the Venly SDK (execute for each call made with any of the Venly APIs)
UVyBeamableProxy::Get()->OnExecuteRequest.BindLambda([this](const FString& Request, const FVyOnExecuteProviderRequestComplete& OnComplete) {
//Initialize the Microservice Request Data
auto microserviceRequest = UVenlyProviderServiceMsExecuteRequest::Make(Request, this, {});
auto requestContext = FBeamRequestContext{};
auto handler = FOnVenlyProviderServiceMsExecuteFullResponse{};
//Handle the Microservice Response
handler.BindLambda([OnComplete](FVenlyProviderServiceMsExecuteFullResponse response) {
//Notify Venly that a Response is received
FVyBeamableMicroserviceResponse MicroserviceResponse{};
//Set the Response 'Success' property
MicroserviceResponse.Success = response.State == EBeamFullResponseState::RS_Success;
//Set the Response 'Result' or 'Error' property
if (MicroserviceResponse.Success) MicroserviceResponse.Result = response.SuccessData->Value;
else MicroserviceResponse.Error = response.ErrorData;
//Invoke OnComplete
OnComplete.ExecuteIfBound(MicroserviceResponse);
});
//Call the Microservice (Execute)
UBeamVenlyProviderServiceMsApi::GetSelf()->CPP_Execute(FUserSlot{ TEXT("Player0") }, microserviceRequest, handler, requestContext, {}, this);
});
}
//...
Updated 10 months ago