Deploy an Ethereum contract
This is a guide with a very simple smart contract example.
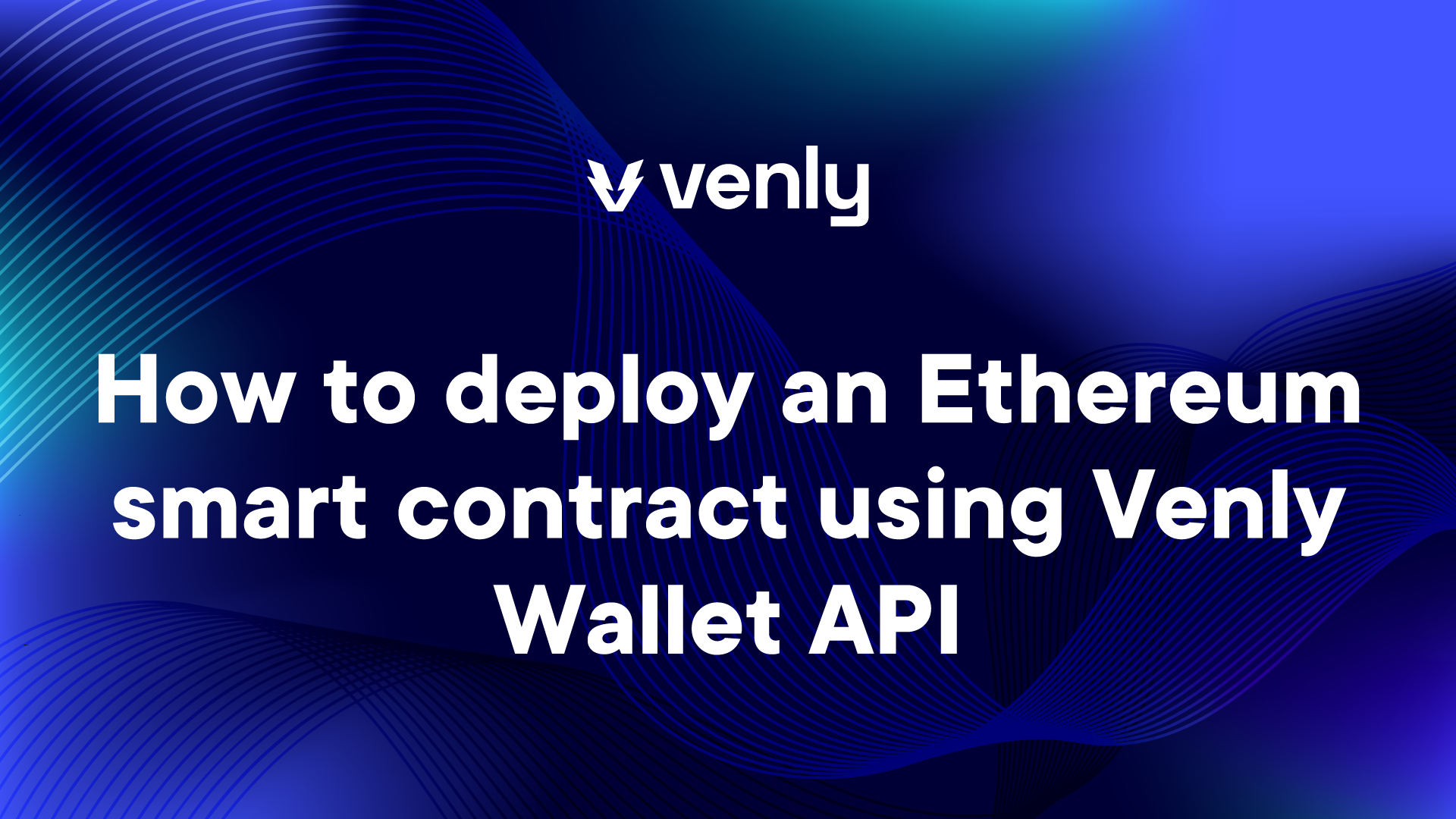
Deploying a smart contract
A smart contract can be deployed using the existing endpoints/functions from Venly
Steps to follow in order to deploy the contract:
1.Develop the solidity contract
Below is a simple Solidity smart contract that you can deploy on the Ethereum blockchain. This contract is a basic example of a token contract that allows you to create
There are several tools and methods available for compiling Solidity code, and I'll provide an example using Remix, a web-based IDE.
Open Remix in your web browser:
// SPDX-License-Identifier: MIT
pragma solidity >=0.4.0 <0.7.0;
contract SimpleStorage {
uint storedData;
function set(uint x) public {
storedData = x;
}
function get() public view returns (uint) {
return storedData;
}
}
Write or paste your Solidity code into the editor. (see example above)
In the Solidity Compiler tab on the left sidebar, you can find the compiler version. Choose the appropriate version for your contract.
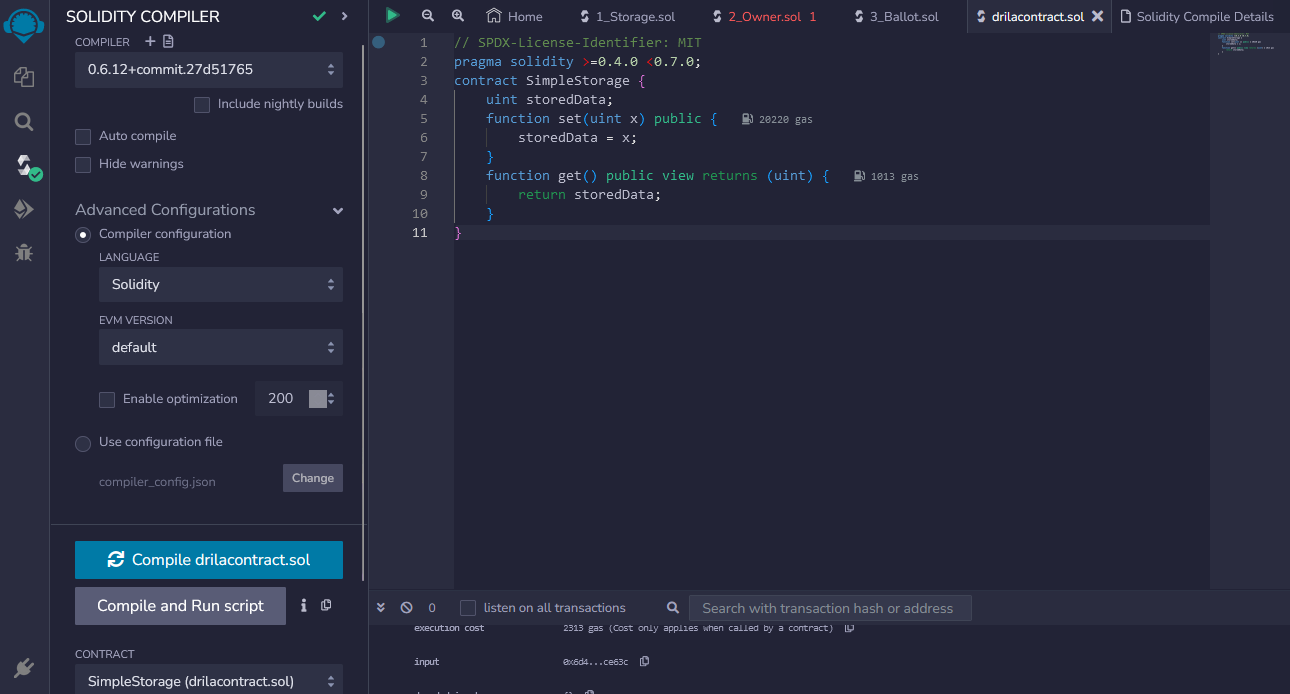
Solidity compiler contract view
Click on the Compile button to compile your contract.
2.Generate the bytecode
To generate the bytecode for a developed Solidity contract, you need to compile the Solidity code into Ethereum Virtual Machine (EVM) bytecode.
After compilation, you will see a list of compiled artifacts including the bytecode. Look for your contract name under the Contracts section, and you'll find the bytecode under BYTECODE.
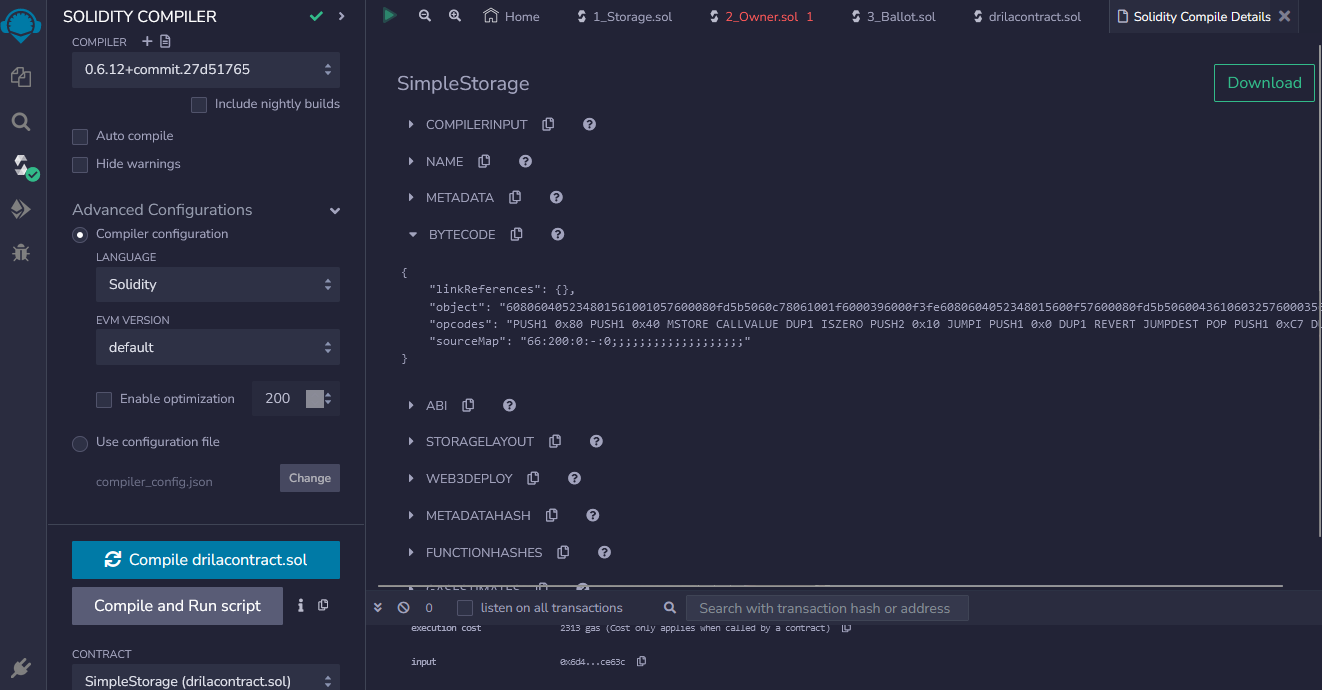
Solidity Compiler Bytecode
Bytecode in this case: 608060405234801561001057600080fd5b5060c78061001f6000396000f3fe6080604052348015600f57600080fd5b506004361060325760003560e01c806360fe47b11460375780636d4ce63c146062575b600080fd5b606060048036036020811015604b57600080fd5b8101908080359060200190929190505050607e565b005b60686088565b6040518082815260200191505060405180910390f35b8060008190555050565b6000805490509056fea264697066735822122018e873e978df16c207f8f6ed18612b17e2c2a70d0916ff978c0755f6a45e26fc64736f6c634300060c0033
3.Deploy the smart contract
To deploy the smart contract you will need to execute a native token transfer where the to field is empty, the value is 0 and the data contains the bytecode see example:
{
"pincode": "123456",
"transactionRequest": {
"type": "TRANSFER",
"walletId": "d7d10358-c36b-495b-9c1c-77237505f5d0",
"value": "0",
"secretType": "ETHEREUM",
"data": "608060405234801561001057600080fd5b5060c78061001f6000396000f3fe6080604052348015600f57600080fd5b506004361060325760003560e01c806360fe47b11460375780636d4ce63c146062575b600080fd5b606060048036036020811015604b57600080fd5b8101908080359060200190929190505050607e565b005b60686088565b6040518082815260200191505060405180910390f35b8060008190555050565b6000805490509056fea264697066735822122018e873e978df16c207f8f6ed18612b17e2c2a70d0916ff978c0755f6a45e26fc64736f6c634300060c0033" // bytecode from step 3
}
}
The response from this request gives us the TX Hash which can be used to check in the block explorer if the contract has been deployed:
{
"success": true,
"result": {
"id": "d7e3f894-4a6b-433d-93b0-f9f2e1972ea3",
"transactionHash": "0x14011289f295db9a46ea7f030e140737a7ea835095171d6e69050e553a0d03a9"
}
}
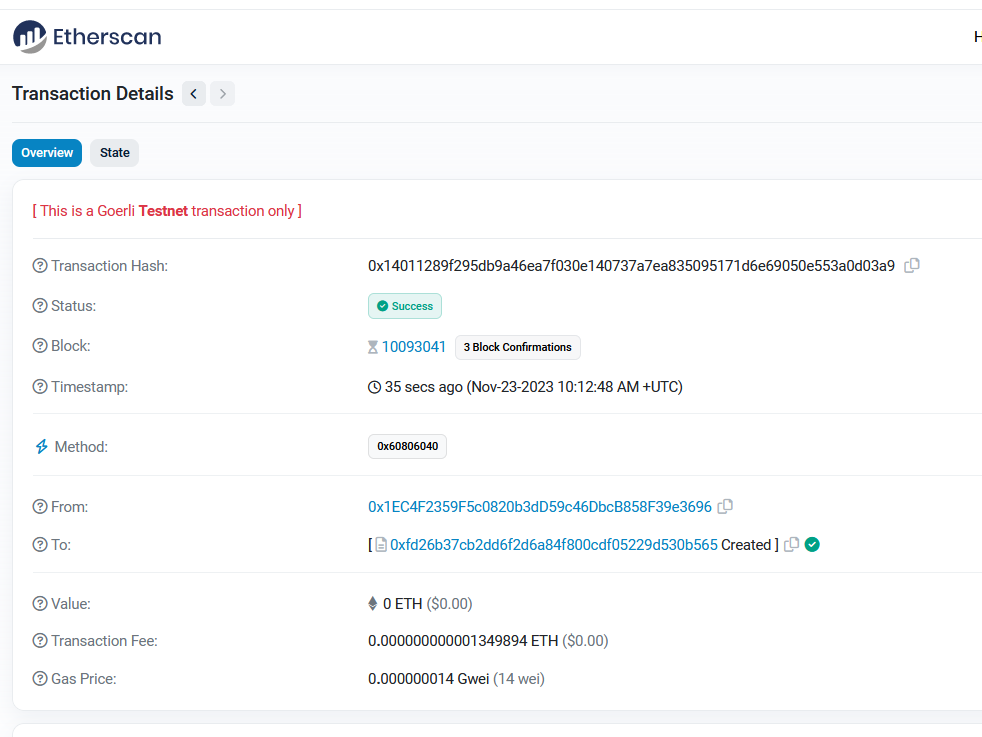
Transaction details in blockchain explorer
https://goerli.etherscan.io/tx/0x14011289f295db9a46ea7f030e140737a7ea835095171d6e69050e553a0d03a9
The wallet executing the native token transfer must have ETH to pay for the gas, if you do not have eth in the wallet, you can add some from the faucets.
Updated 3 days ago