Getting started
Covering the basics from authentication to minting your first NFT.
What we cover in this guide
In this guide, we will explain how to authenticate to our API service and create your first NFT. We will walk you through several endpoints to help you get started. We will cover the following topics:
- Authentication
- Creating a Contract
- Checking Contract Status
- Creating Token-type (NFT template)
- Checking Token-type Status
- Minting NFT
- Checking NFT Mint Status
Getting Started Flow
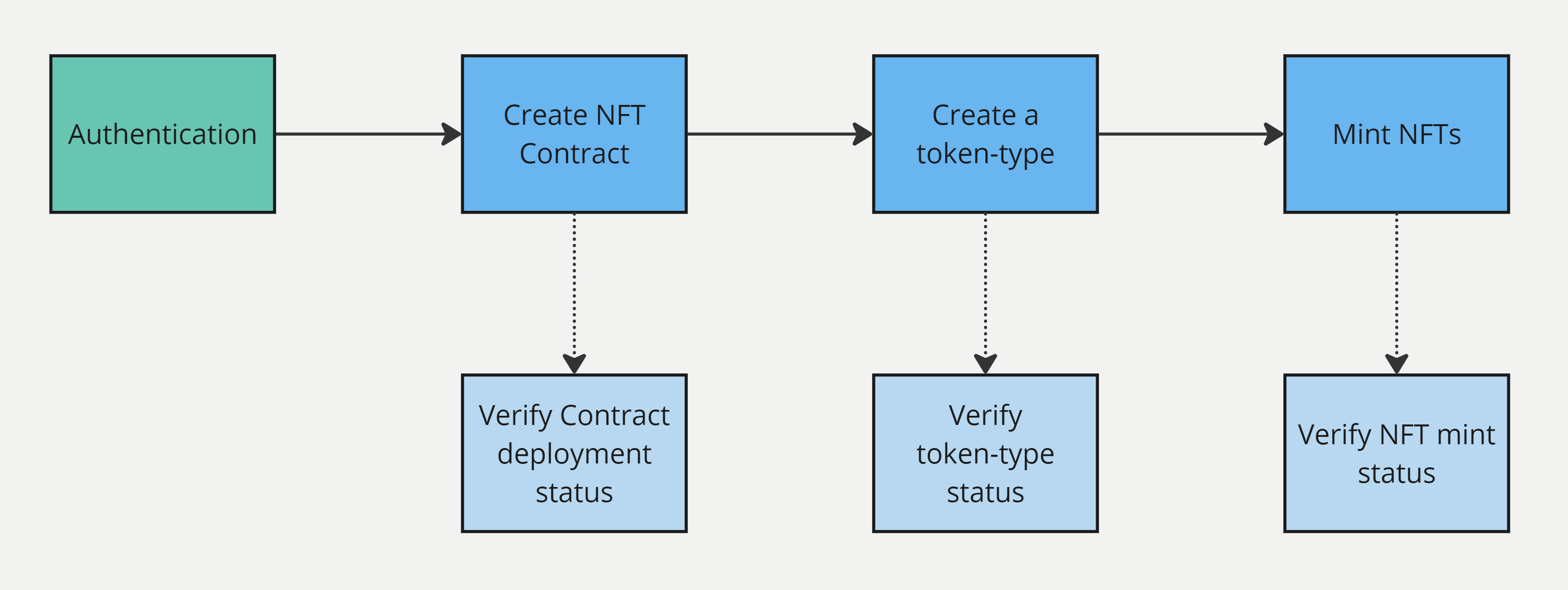
Getting Started flow for NFT API
NFT API Tutorial Video
Prerequisites
- You need a Venly business account, if you don't have one, click here to register in our Developer Portal, or follow our step-by-step guide, Getting Started with Venly.
- You need your Client ID and Client Secret which can be obtained from the Portal as shown below.
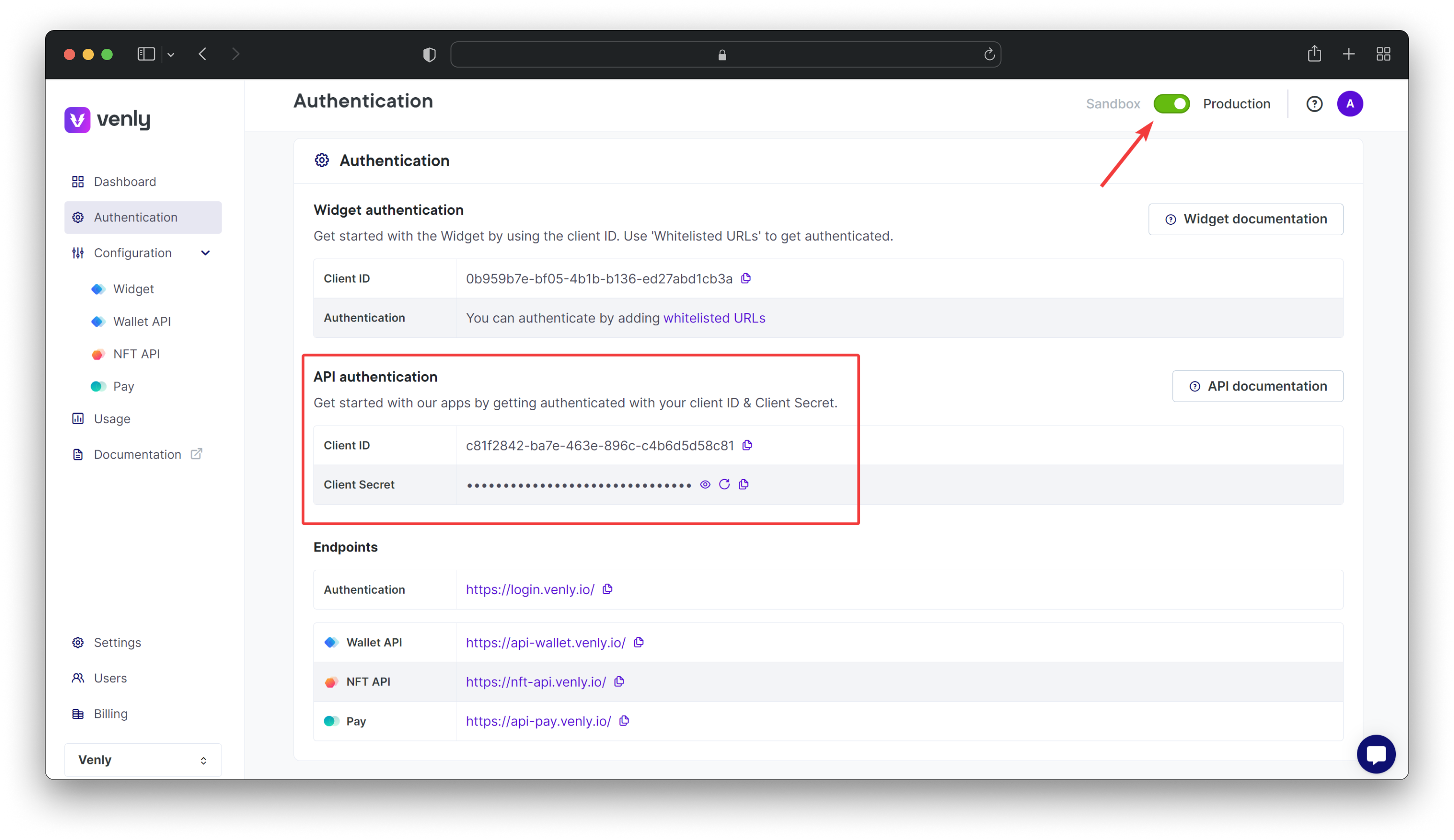
Access Credentials - Developer Portal
All the API calls for this guide run on a sandbox environment. You can test and experiment with API calls without causing any harm to the actual blockchain or data.
There are several ways to run API calls, but for this guide, you can use the API-Reference or Postman to execute the different endpoints.
1. Authenticating
You will need your access credentials (Client ID and Client Secret) to obtain a bearer token
and authorize all API calls. These credentials are necessary for authentication purposes.
- Learn how to retrieve a bearer token and authenticate API calls.
- Please note that the base path for all NFT API endpoints is
https://nft-api-sandbox.venly.io
2. Creating a Contract
Let's start by defining your first contract. This contract will represent a collection for the NFTs. We will create a contract on the Polygon (MATIC) testnet chain.
As the API calls are running in a sandbox environment, the contract will be created on a testnet chain. In this example we will create an NFT contract on the MATIC testnet chain.
Request Endpoint: reference
POST /api/v3/erc1155/contracts/deployments
Request Body:
Parameter | Description | Type | Required |
---|---|---|---|
name | The name of your NFT collection/contract | String | ✅ |
description | The description of your NFT collection/contract | String | ✅ |
image | The image URL for your NFT collection/contract that will be displayed | String | ✅ |
externalUrl | This can be any link such as a link to your website, landing page, etc. | String | ✅ |
chain | This is the blockchain on which you want to create the contract on | String | ✅ |
{
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"externalUrl": "https://www.venly.io/",
"chain": "MATIC"
}
Response Body:
Save the
result.id
from the response body. This is the Deployment ID used to track the status of the contract creation request.
{
"success": true,
"result": {
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"id": "5ad5b26d-77d0-46ee-aa4d-569878b74b58",
"chain": "MATIC",
"symbol": "VENFCO",
"externalUrl": "https://www.venly.io/",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"media": [],
"transactionHash": "0xa0b413f2584a38bab757f7a25def86efcf43eb19adf3dba529b646f2811f8a96",
"status": "PENDING",
"storage": {
"type": "cloud",
"location": "https://metadata-staging.venly.io/metadata/contracts/67822"
},
"contractUri": "https://metadata-staging.venly.io/metadata/contracts/67822",
"royalties": {},
"external_link": "https://www.venly.io/"
}
}
3. Check the status of the contract/collection
This endpoint is used to check the deployment status of a contract/collection. The {deploymentId}
in the path is for tracking the status of contract creation. It is in the response body of the create contract/collection endpoint as result.id
.
Request Endpoint: reference
GET /api/v3/erc1155/contracts/deployments/{deploymentId}
Example Request
GET /api/v3/erc1155/contracts/deployments/5ad5b26d-77d0-46ee-aa4d-569878b74b58
Response Body
In the response body look for the result.
status
parameter. It can have three possible values:
SUCCEEDED
PENDING
FAILED
{
"success": true,
"result": {
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"id": "5ad5b26d-77d0-46ee-aa4d-569878b74b58",
"chain": "MATIC",
"symbol": "VENFCO",
"externalUrl": "https://www.venly.io/",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"media": [],
"transactionHash": "0xa0b413f2584a38bab757f7a25def86efcf43eb19adf3dba529b646f2811f8a96",
"status": "SUCCEEDED",
"storage": {
"type": "cloud",
"location": "https://metadata-staging.venly.io/metadata/contracts/67822"
},
"contractUri": "https://metadata-staging.venly.io/metadata/contracts/67822",
"external_link": "https://www.venly.io/"
}
}
4. Creating Token-type
Next, we will create a token-type which serves as a template for minting NFTs. This means you just have to define the NFT template once with parameters like its name, image, attributes, etc, and then you can mint multiple NFTs directly to your end-users wallets.
Defining token types helps structure the information such as attributes and animationUrls.
Request Endpoint: reference
POST /api/v3/erc1155/token-types/creations
Request Body:
Parameter | Description | Type | Required |
---|---|---|---|
chain | The blockchain of the contract | String | ✅ |
contractAddress | The contract address under which you want to create the token-type | String | ✅ |
creations | An array of objects that can define one or multiple token-type details | Array of objects | ✅ |
creations.name | The name of the token-type | String | ✅ |
creations.description | The description of the token-type | String | ❌ |
creations.image | The image URL for the token-type that will be displayed | String | ❌ |
{
"chain": "MATIC",
"contractAddress": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"creations": [
{
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg"
}
]
}
Response Body:
- Save the
result.creations.id
from the response body. This is the token Creation ID and it's used to track the status of the token-type creation request.- The
status
attribute indicates the token-type creation status.
{
"success": true,
"result": {
"creations": [
{
"id": "92ebfbb7-df9a-4228-9b57-6614315fc896",
"status": "PENDING",
"tokenTypeId": 1,
"metadata": {
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imagePreview": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imageThumbnail": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"animationUrls": [],
"attributes": [
{
"type": "system",
"name": "tokenTypeId",
"value": "1",
"traitType": "Token Type ID",
"trait_type": "Token Type ID"
}
],
"contract": {
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"name": "Venly NFT Collection",
"symbol": "VENFCO",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"imageUrl": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"image_url": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"description": "This collection contains Venly NFTs.",
"externalLink": "https://www.venly.io/",
"external_link": "https://www.venly.io/",
"externalUrl": "https://www.venly.io/",
"external_url": "https://www.venly.io/",
"media": [],
"type": "ERC_1155"
},
"fungible": false
}
}
]
}
}
5. Check the status of token-type
This endpoint is used to check the status of token-type creation. The {creationId}
in the path is for tracking the status of token-type creation. It is in the response body of the create token-type endpoint as result.id
.
Request Endpoint: reference
GET /api/v3/erc1155/token-types/creations/{creationId}
Example Request
GET /api/v3/erc1155/token-types/creations/92ebfbb7-df9a-4228-9b57-6614315fc896
Response Body
In the response body look for the result.
status
parameter. It can have three possible values:
SUCCEEDED
PENDING
FAILED
{
"success": true,
"result": {
"id": "ff81e5e5-68eb-4ba1-949d-b82d9adf30ad",
"status": "SUCCEEDED",
"transactionHash": "0x892a5740a0a2ae77a79218beb4891411b3f9e12fb54c59f0e044b6157e22ba66",
"tokenTypeId": 2,
"mints": [],
"metadata": {
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imagePreview": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imageThumbnail": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"animationUrls": [],
"attributes": [
{
"type": "system",
"name": "tokenTypeId",
"value": "2",
"traitType": "Token Type ID",
"trait_type": "Token Type ID"
}
],
"contract": {
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"name": "Venly NFT Collection",
"symbol": "VENFCO",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"imageUrl": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"image_url": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"description": "This collection contains Venly NFTs.",
"externalLink": "https://www.venly.io/",
"external_link": "https://www.venly.io/",
"externalUrl": "https://www.venly.io/",
"external_url": "https://www.venly.io/",
"media": [],
"type": "ERC_1155"
},
"fungible": false
}
}
}
6. Minting an NFT
Request Endpoint: reference
POST /api/v3/erc1155/contracts/deployments
Request Body:
Parameter | Param Type | Description | Type | Required |
---|---|---|---|---|
contractAddress | Body | The contract address | String | ✅ |
chain | Body | The blockchain of the contract | String | ✅ |
tokenTypeId | Body | This is the ID of the token-type. You can get it from the response body when you create a token-type. | String | ✅ |
destinations | Body | The array which includes all the wallet addresses and the number of NFTs to mint per wallet address | Array of objects | ✅ |
destinations.address | Body | The wallet address to mint and send the NFT | String | ✅ |
destinations.amount | Body | The number of NFTs you want to mint and send | Integer | ✅ |
{
"contractAddress": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"chain": "MATIC",
"tokenTypeId": "2",
"destinations": [
{
"address": "0x9A9F6A560bBdCFbbb014303fc817D1e5EeaA3AE2",
"amount": 1
}
]
}
- The destinations.
address
can be either a wallet address or an email address.- NOTE: The
contractAddress
,chain
(blockchain), and thetokenTypeId
are defined in the request body.
Response Body:
- Under the
mints
array, you can find theid
(mintId) for each minted NFT listed with the wallet address. This uniqueid
can be used to track the status of the mint request.- The
status
attribute indicates the on-chain token mint status.
{
"success": true,
"result": {
"mints": [
{
"id": "e9943c23-25bb-4de1-9679-9b1a880c31df",
"createdOn": "2024-09-25T11:10:32.393465624",
"status": "PENDING",
"destination": {
"address": "0x9A9F6A560bBdCFbbb014303fc817D1e5EeaA3AE2",
"amount": 1
}
}
],
"metadata": {
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imagePreview": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imageThumbnail": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"animationUrls": [],
"attributes": [
{
"type": "system",
"name": "tokenTypeId",
"value": "2",
"traitType": "Token Type ID",
"trait_type": "Token Type ID"
}
],
"contract": {
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"name": "Venly NFT Collection",
"symbol": "VENFCO",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"imageUrl": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"image_url": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"description": "This collection contains Venly NFTs.",
"externalLink": "https://www.venly.io/",
"external_link": "https://www.venly.io/",
"externalUrl": "https://www.venly.io/",
"external_url": "https://www.venly.io/",
"media": [],
"type": "ERC_1155"
},
"fungible": false
}
}
}
7. Check the status of NFT mints
This endpoint is used to check the status of NFT mints. The {mintId}
in the path is for tracking the status of the NFT mint. It is in the response body of the Mint Fungible or Non-Fungible Tokens endpoint as result.mints.id
.
Request Endpoint: reference
GET /api/v3/erc1155/tokens/mints/{mintId}
Example Request
GET /api/v3/erc1155/tokens/mints/e9943c23-25bb-4de1-9679-9b1a880c31df
Response Body
- You can get the
tokenId
in the response body.- In the response body look for the result.
status
parameter. It can have three possible values:
SUCCEEDED
PENDING
FAILED
{
"success": true,
"result": {
"id": "e9943c23-25bb-4de1-9679-9b1a880c31df",
"tokenId": 3,
"createdOn": "2024-09-25T11:10:32.393466",
"status": "SUCCEEDED",
"transactionHash": "0x0f0070ffa177822a164fad8d9d6d3a0268ed1b717975e3901d02a8bb3623981a",
"destination": {
"address": "0x9A9F6A560bBdCFbbb014303fc817D1e5EeaA3AE2",
"amount": 1
},
"metadata": {
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imagePreview": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imageThumbnail": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"animationUrls": [],
"attributes": [
{
"type": "system",
"name": "tokenTypeId",
"value": "2",
"traitType": "Token Type ID",
"trait_type": "Token Type ID"
},
{
"type": "property",
"name": "mintNumber",
"value": "1",
"traitType": "Mint Number",
"trait_type": "Mint Number"
}
],
"contract": {
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"name": "Venly NFT Collection",
"symbol": "VENFCO",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"imageUrl": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"image_url": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"description": "This collection contains Venly NFTs.",
"externalLink": "https://www.venly.io/",
"external_link": "https://www.venly.io/",
"externalUrl": "https://www.venly.io/",
"external_url": "https://www.venly.io/",
"media": [],
"type": "ERC_1155"
},
"fungible": false
}
}
}
View your NFT on testnet
Learn how to view your NFT on sandbox/testnet.

Schedule a demo with our team to explore tailored solutions or dive in and start building right away on our portal.
Updated 1 day ago