Simple NFT Application
Your First NFT Application with Venly
Intro
Here is an example of a basic NFT application. Venly can help you mitigate challenges around user onboarding for non-crypto-savvy users. Using Venly's multi-tiered services, you only need to interface with one service to create a seamless end-to-end experience.
The technical architecture outlined below shows you how to structure your backend to accommodate a "crypto-less" flow from your user's perspective and settle user flows on the blockchain.
In this example, we use the Wallet API and the NFT API. Via the Wallet API, we can create blockchain wallets for all users, and the NFT API allows us to create a digital asset in the form of an NFT and transfer it to the wallet we created for the user. All this without exposing any blockchain element to the end customer.
If you also want to implement a payment solution in your architecture, then our PAY solution would be a good fit. It allows you to sell NFT and regular products via the most common payment rails.
With only 11 API calls, you can:
- create a user
- create a wallet
- create an NFT contract
- mint NFTs
Services used in this example
- Wallet API - Used for the wallet creation
- NFT API - Used for the creation and transfer of NFTs
- PAY API - Acts as the payment provider for selling NFTs (optional)
Guides we recommend to read
- Authentication - Requesting and retrieving the bearer token
- Create a user - Create a user account for each of your end customers
- Create a wallet- Create a wallet for your users
- Mint an NFT - Create, mint and transfer an NFT
Complete Flow Diagram
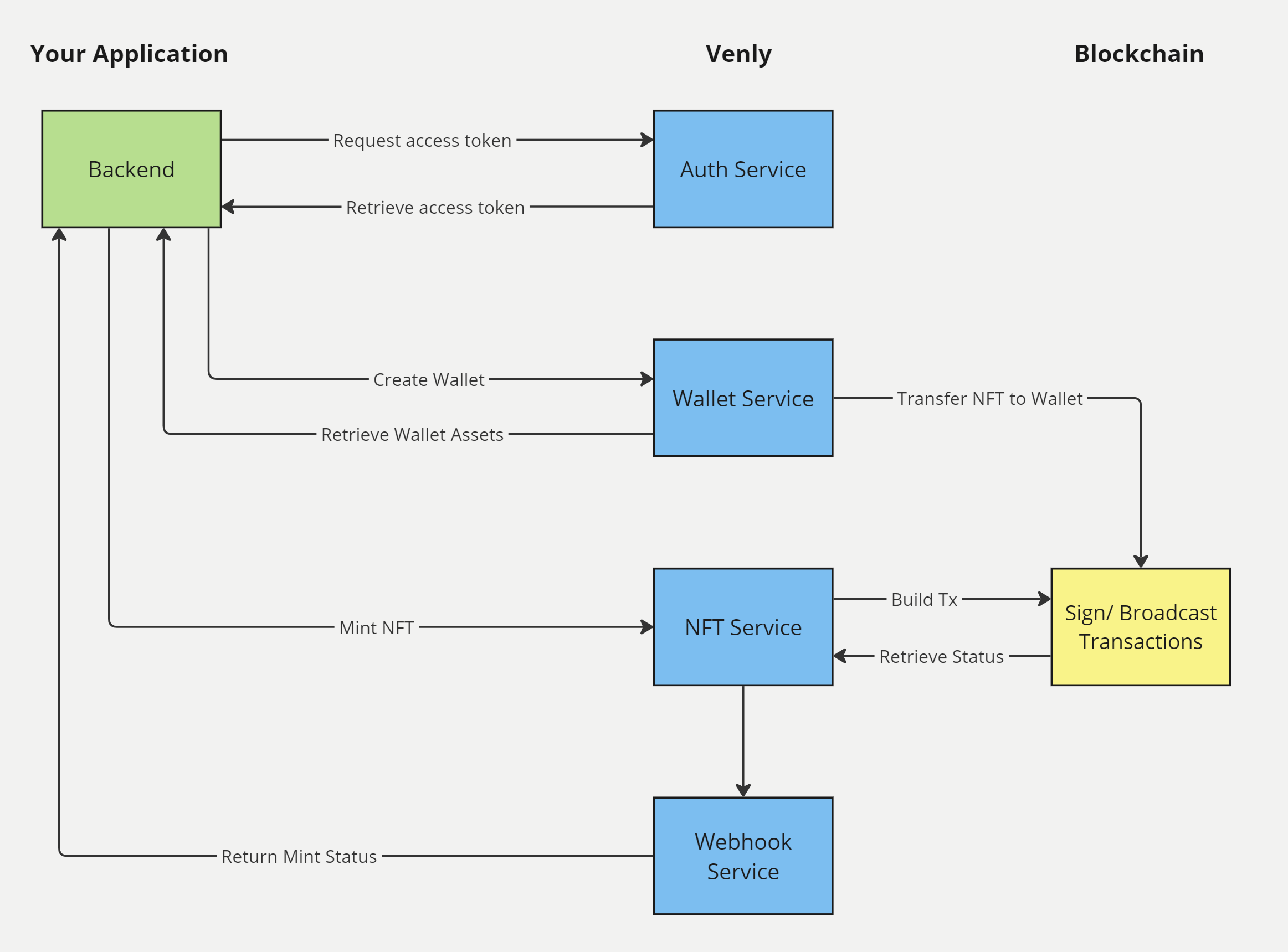
Complete NFT Application flow - component overview
Complete Endpoints
Now we will go into detail and look at the endpoints you need to execute to authenticate, create a user, and mint NFTs. We will break this down into three parts.
First, we will learn how to authenticate, then how to create a user and a wallet for that user, and finally we will learn how to mint an NFT to the user's wallet address.
1. Authenticating
You will need your access credentials (Client ID and Client Secret) from the Portal, to obtain a bearer token
and authorize all API calls. These credentials are necessary for authentication purposes. The bearer token is to be passed in the header of each API call.
- Learn how to retrieve a bearer token and authenticate API calls.
- Please note that the base path for all Wallet API endpoints in this guide specifically is
https://api-wallet-sandbox.venly.io
, which is the sandbox environment.
1.1 Video Guide
2. Creating a User and their Wallet
2.1. Creating a User
Let's look at how to create a user. Each user created can represent one of your end-users.
Read more about User Management.
Use the following endpoint to create a user:
Request Endpoint: reference
POST /api/users
Parameter | Param Type | Description | Data Type | Mandatory |
---|---|---|---|---|
reference | Body | A free-form text to specify a reference for the user you create. It can help group specific users. | String | ❌ |
Request Body:
{
"reference": "Test User"
}
Response Body:
result.id
: This is the User ID, of your user.
{
"success": true,
"result": {
"id": "d5eabcf2-f9f0-4341-bf89-807420bb051c",
"reference": "Test User",
"createdAt": "2024-10-02T15:44:42.551793919"
}
}
2.2. Creating a signing method for your user
Next, we're going to create a PIN
signing method for the user we just created. There are other signing methods as well, but the very first one should be PIN
. Signing methods allow your users to access and interact with their wallets and perform transactions. Each user can access their wallets using their own signing method.
Read more about Signing Methods.
Use the following endpoint to create a signing method for your user.
Request Endpoint: reference
POST /api/users/{userId}/signing-methods
Parameter | Param Type | Description | Data Type | Mandatory |
---|---|---|---|---|
{userId} | Path | The ID of the user for whom to create the signing method for | String | ✅ |
type | Body | The type of signing method to create for this user. Allowed values: PIN, BIOMETRIC, EMERGENCY_CODE | String | ✅ |
value | Body | The value of the signing method. For PIN , the value should be 6 digits. For EMERGENCY_CODE , the value should be 25 characters, or you can leave it blank to autogenerate the code with the correct entropy. | String | ❌ |
Request Body:
{
"type": "PIN",
"value": "123456"
}
Response Body:
result.id
: This is the Signing Method ID, of your user.- Each user has a unique signing method ID
{
"success": true,
"result": {
"id": "bf1200ce-d117-4a06-a029-6d99080c6358",
"type": "PIN",
"incorrectAttempts": 0,
"remainingAttempts": 10,
"hasMasterSecret": true
}
}
2.3. Creating a Wallet
The next step is creating a wallet for your user. We will include the userId
in the request body, and this way the wallet will be created and linked to the specific user. The user can then access the wallet with their signing method.
Since you currently use the API calls in a sandbox environment, the wallets will be created on a testnet chain. In this example, we will create the wallet on the Polygon (MATIC) Blockchain.
Explore testnet chains further.
Use the following endpoint to create a wallet for your user:
Request Endpoint: reference
POST /api/wallets
Parameter | Param Type | Value | Description | Example Value |
---|---|---|---|---|
Signing-Method | Header | id:value | id : This is the ID of the user's signing methodvalue : This is the value of the user's signing method | c992826c-6311-4896-9c74-8a5f1644f9c7:123456 |
Parameter | Description | Type | Required |
---|---|---|---|
secretType | Specifies the blockchain on which you wish to create your wallet | String | ✅ |
userId | The ID of the user you want to link to this wallet. | String | ❌ |
Request Body:
{
"secretType": "MATIC",
"userId": "ddf04a49-9c38-4326-acc7-02529ba05a78"
}
Response Body:
Save the following parameters from the response body. They will be used later in the guide.
Parameter | Description |
---|---|
result.id | This is the Wallet ID. It is utilized to uniquely identify the wallet. |
result.address | This is the public wallet address. It will be used when we mint NFTs. |
{
"success": true,
"result": {
"id": "19590dc9-28af-417b-bc66-549e726bf91f",
"address": "0xa1f04C769155195D83c7f16bC6B9540139C80b2A",
"walletType": "API_WALLET",
"secretType": "MATIC",
"createdAt": "2023-12-07T10:21:25.114751052",
"archived": false,
"description": "Wise Iguana",
"primary": false,
"hasCustomPin": false,
"userId": "ddf04a49-9c38-4326-acc7-02529ba05a78",
"custodial": false,
"balance": {
"available": true,
"secretType": "MATIC",
"balance": 0,
"gasBalance": 0,
"symbol": "POL",
"gasSymbol": "POL",
"rawBalance": "0",
"rawGasBalance": "0",
"decimals": 18
}
}
}
You have successfully created a MATIC wallet for your user 🎉.
Next let's look at how to mint NFTs!
3. Minting NFTs
By now you should have an empty wallet created for your user. Next, we will learn how to easily mint NFTs to that user's wallet address. As you created a MATIC wallet, we will be creating an NFT contract on the MATIC chain and then mint the NFTs.
3.1 Creating a Contract
Let's start by defining your first contract. This contract will represent a collection for the NFTs. We will create a contract on the Polygon (MATIC) testnet chain.
Request Endpoint: reference
POST /api/v3/erc1155/contracts/deployments
Request Body:
Parameter | Description | Type | Required |
---|---|---|---|
name | The name of your NFT collection/contract | String | ✅ |
description | The description of your NFT collection/contract | String | ✅ |
image | The image URL for your NFT collection/contract that will be displayed | String | ✅ |
externalUrl | This can be any link such as a link to your website, landing page, etc. | String | ✅ |
chain | This is the blockchain on which you want to create the contract on | String | ✅ |
{
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"externalUrl": "https://www.venly.io/",
"chain": "MATIC"
}
Response Body:
Save the
result.id
from the response body. This is the Deployment ID used to track the status of the contract creation request.
{
"success": true,
"result": {
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"id": "5ad5b26d-77d0-46ee-aa4d-569878b74b58",
"chain": "MATIC",
"symbol": "VENFCO",
"externalUrl": "https://www.venly.io/",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"media": [],
"transactionHash": "0xa0b413f2584a38bab757f7a25def86efcf43eb19adf3dba529b646f2811f8a96",
"status": "PENDING",
"storage": {
"type": "cloud",
"location": "https://metadata-staging.venly.io/metadata/contracts/67822"
},
"contractUri": "https://metadata-staging.venly.io/metadata/contracts/67822",
"royalties": {},
"external_link": "https://www.venly.io/"
}
}
3.2 Check the status of the contract/collection
This endpoint is used to check the deployment status of a contract/collection. The {deploymentId}
in the path is for tracking the status of contract creation. It is in the response body of the create contract/collection endpoint as result.id
.
Request Endpoint: reference
GET /api/v3/erc1155/contracts/deployments/{deploymentId}
Example Request
GET /api/v3/erc1155/contracts/deployments/5ad5b26d-77d0-46ee-aa4d-569878b74b58
Response Body
- In the response body, the result.
status
parameter is SUCCEEDED, indicating the contract was deployed on-chain.- Save the result.
address
parameter. It is the contract address and it will be used when minting NFTs.
{
"success": true,
"result": {
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"id": "5ad5b26d-77d0-46ee-aa4d-569878b74b58",
"chain": "MATIC",
"symbol": "VENFCO",
"externalUrl": "https://www.venly.io/",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"media": [],
"transactionHash": "0xa0b413f2584a38bab757f7a25def86efcf43eb19adf3dba529b646f2811f8a96",
"status": "SUCCEEDED",
"storage": {
"type": "cloud",
"location": "https://metadata-staging.venly.io/metadata/contracts/67822"
},
"contractUri": "https://metadata-staging.venly.io/metadata/contracts/67822",
"external_link": "https://www.venly.io/"
}
}
3.3 Creating Token-type
Next, we will create a token-type which serves as a template for minting NFTs. This means you just have to define the NFT template once with parameters like its name, image, attributes, etc, and then you can mint multiple NFTs directly to your user's wallets.
Defining token types helps structure the information such as attributes and animationUrls.
Request Endpoint: reference
POST /api/v3/erc1155/token-types/creations
Request Body:
Parameter | Description | Type | Required |
---|---|---|---|
chain | The blockchain of the contract | String | ✅ |
contractAddress | The contract address under which you want to create the token-type | String | ✅ |
creations | An array of objects that can define one or multiple token-type details | Array of objects | ✅ |
creations.name | The name of the token-type | String | ✅ |
creations.description | The description of the token-type | String | ❌ |
creations.image | The image URL for the token-type that will be displayed | String | ❌ |
{
"chain": "MATIC",
"contractAddress": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"creations": [
{
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg"
}
]
}
Response Body:
- Save the
result.creations.id
from the response body. This is the token Creation ID and it's used to track the status of the token-type creation request.- The
status
attribute indicates the token-type creation status.
{
"success": true,
"result": {
"creations": [
{
"id": "92ebfbb7-df9a-4228-9b57-6614315fc896",
"status": "PENDING",
"tokenTypeId": 2,
"metadata": {
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imagePreview": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imageThumbnail": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"animationUrls": [],
"attributes": [
{
"type": "system",
"name": "tokenTypeId",
"value": "1",
"traitType": "Token Type ID",
"trait_type": "Token Type ID"
}
],
"contract": {
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"name": "Venly NFT Collection",
"symbol": "VENFCO",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"imageUrl": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"image_url": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"description": "This collection contains Venly NFTs.",
"externalLink": "https://www.venly.io/",
"external_link": "https://www.venly.io/",
"externalUrl": "https://www.venly.io/",
"external_url": "https://www.venly.io/",
"media": [],
"type": "ERC_1155"
},
"fungible": false
}
}
]
}
}
3.4 Check the status of token-type
This endpoint is used to check the status of token-type creation. The {creationId}
in the path is for tracking the status of token-type creation. It is in the response body of the create token-type endpoint as result.id
.
Request Endpoint: reference
GET /api/v3/erc1155/token-types/creations/{creationId}
Example Request
GET /api/v3/erc1155/token-types/creations/92ebfbb7-df9a-4228-9b57-6614315fc896
Response Body
- In the response body, the result.
status
parameter is SUCCEEDED, indicating the token-type was created.- Save the result.
tokenTypeId
parameter. It will be used when minting NFTs.
{
"success": true,
"result": {
"id": "ff81e5e5-68eb-4ba1-949d-b82d9adf30ad",
"status": "SUCCEEDED",
"transactionHash": "0x892a5740a0a2ae77a79218beb4891411b3f9e12fb54c59f0e044b6157e22ba66",
"tokenTypeId": 2,
"mints": [],
"metadata": {
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imagePreview": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imageThumbnail": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"animationUrls": [],
"attributes": [
{
"type": "system",
"name": "tokenTypeId",
"value": "2",
"traitType": "Token Type ID",
"trait_type": "Token Type ID"
}
],
"contract": {
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"name": "Venly NFT Collection",
"symbol": "VENFCO",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"imageUrl": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"image_url": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"description": "This collection contains Venly NFTs.",
"externalLink": "https://www.venly.io/",
"external_link": "https://www.venly.io/",
"externalUrl": "https://www.venly.io/",
"external_url": "https://www.venly.io/",
"media": [],
"type": "ERC_1155"
},
"fungible": false
}
}
}
3.5 Minting NFTs
Finally, let's look at how to mint NFTs to the user's wallet address. By now you should have:
- User's wallet address:
0xa1f04C769155195D83c7f16bC6B9540139C80b2A
- Wallet ID:
19590dc9-28af-417b-bc66-549e726bf91f
- NFT contract address:
0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3
- Token-type ID:
2
Request Endpoint: reference
POST /api/v3/erc1155/contracts/deployments
Request Body:
Parameter | Param Type | Description | Type | Required |
---|---|---|---|---|
contractAddress | Body | The contract address | String | ✅ |
chain | Body | The blockchain of the contract | String | ✅ |
tokenTypeId | Body | This is the ID of the token-type. You can get it from the response body when you create a token-type. | String | ✅ |
destinations | Body | The array which includes all the wallet addresses and the number of NFTs to mint per wallet address | Array of objects | ✅ |
destinations.address | Body | The wallet address or email address to mint and send the NFT | String | ✅ |
destinations.amount | Body | The number of NFTs you want to mint and send | Integer | ✅ |
{
"contractAddress": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"chain": "MATIC",
"tokenTypeId": "2",
"destinations": [
{
"address": "0xa1f04C769155195D83c7f16bC6B9540139C80b2A",
"amount": "5"
}
]
}
Response Body:
- Under the
mints
array, you can find theid
(mintId) for each minted NFT listed with the wallet address. This uniqueid
(mintId) can be used to track the status of the mint request.- The
status
attribute indicates the on-chain token mint status.
{
"success": true,
"result": {
"mints": [
{
"id": "39fb5210-427d-4fc2-93cf-2e4476e2e457",
"createdOn": "2024-10-02T12:47:26.227696583",
"status": "PENDING",
"destination": {
"address": "0xa1f04C769155195D83c7f16bC6B9540139C80b2A",
"amount": 1
}
},
{
"id": "95ba44d2-a9ad-462d-91b1-af7e5542dbd1",
"createdOn": "2024-10-02T12:47:26.22994071",
"status": "PENDING",
"destination": {
"address": "0xa1f04C769155195D83c7f16bC6B9540139C80b2A",
"amount": 1
}
},
{
"id": "a9d472b0-9fbf-4862-a74a-23484fd9017f",
"createdOn": "2024-10-02T12:47:26.231049558",
"status": "PENDING",
"destination": {
"address": "0xa1f04C769155195D83c7f16bC6B9540139C80b2A",
"amount": 1
}
},
{
"id": "f7024a96-32a9-4123-9d15-0f3036d09674",
"createdOn": "2024-10-02T12:47:26.232371192",
"status": "PENDING",
"destination": {
"address": "0xa1f04C769155195D83c7f16bC6B9540139C80b2A",
"amount": 1
}
},
{
"id": "3b75d75f-91f6-4f7d-91b9-95a0c0597327",
"createdOn": "2024-10-02T12:47:26.233422771",
"status": "PENDING",
"destination": {
"address": "0xa1f04C769155195D83c7f16bC6B9540139C80b2A",
"amount": 1
}
}
],
"metadata": {
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imagePreview": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imageThumbnail": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"animationUrls": [],
"attributes": [
{
"type": "system",
"name": "tokenTypeId",
"value": "2",
"traitType": "Token Type ID",
"trait_type": "Token Type ID"
}
],
"contract": {
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"name": "Venly NFT Collection",
"symbol": "VENFCO",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"imageUrl": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"image_url": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"description": "This collection contains Venly NFTs.",
"externalLink": "https://www.venly.io/",
"external_link": "https://www.venly.io/",
"externalUrl": "https://www.venly.io/",
"external_url": "https://www.venly.io/",
"media": [],
"type": "ERC_1155"
},
"fungible": false
}
}
}
3.6 Check the status of NFT mints
This endpoint is used to check the status of NFT mints. The {mintId}
in the path is for tracking the status of the NFT mint. It is in the response body of the Mint Fungible or Non-Fungible Tokens endpoint as result.mints.id
.
Request Endpoint: reference
GET /api/v3/erc1155/tokens/mints/{mintId}
Example Request
GET /api/v3/erc1155/tokens/mints/39fb5210-427d-4fc2-93cf-2e4476e2e457
Response Body
- You can get the
tokenId
in the response body.- In the response body, the result.
status
parameter is SUCCEEDED, indicating the mint was completed on-chain.
{
"success": true,
"result": {
"id": "39fb5210-427d-4fc2-93cf-2e4476e2e457",
"tokenId": 39,
"createdOn": "2024-10-02T12:47:26.227697",
"status": "SUCCEEDED",
"transactionHash": "0x4e39beb176b663233019d7e1de74584c6507a4dcdc1f01ade4ac1d6cb53fa947",
"destination": {
"address": "0xa1f04C769155195D83c7f16bC6B9540139C80b2A",
"amount": 1
},
"metadata": {
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imagePreview": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"imageThumbnail": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/purplevillage.jpeg",
"animationUrls": [],
"attributes": [
{
"type": "system",
"name": "tokenTypeId",
"value": "2",
"traitType": "Token Type ID",
"trait_type": "Token Type ID"
},
{
"type": "property",
"name": "mintNumber",
"value": "2",
"traitType": "Mint Number",
"trait_type": "Mint Number"
}
],
"contract": {
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"name": "Venly NFT Collection",
"symbol": "VENFCO",
"image": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"imageUrl": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"image_url": "https://storage-staging.venly.io/applications/bff93371-db40-4363-9692-4fbe64a34b54/logovenly.png",
"description": "This collection contains Venly NFTs.",
"externalLink": "https://www.venly.io/",
"external_link": "https://www.venly.io/",
"externalUrl": "https://www.venly.io/",
"external_url": "https://www.venly.io/",
"media": [],
"type": "ERC_1155"
},
"fungible": false
}
}
}
3.7 Get NFTs by Wallet ID
Next, we will fetch the NFTs held in a wallet by wallet ID.
Request Endpoint: reference
GET /api/wallets/{walletId}/nonfungibles
Parameter | Param Type | Description | Type | Required |
---|---|---|---|---|
{walletId} | Path | The unique ID of the wallet for which you want to fetch NFTs. It is found in the response body of the create wallet endpoint as result.id | String | ✅ |
Example Request:
GET /api/wallets/19590dc9-28af-417b-bc66-549e726bf91f/nonfungibles
Response Body
The response body shows that your user's wallet has five NFTs!
{
"success": true,
"result": [
{
"id": "43",
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"imageUrl": "https://cdn.simplehash.com/assets/d5364a69e220124da8d406d3f49ee5c7ffbfb2b6ca84d73e3e99878dc28c0b8d.jpg",
"imagePreviewUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA",
"imageThumbnailUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA=s250",
"animationUrls": [],
"fungible": false,
"contract": {
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"symbol": "VENFCO",
"media": [
{
"type": "image",
"value": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
}
],
"type": "ERC_1155",
"verified": false,
"premium": false,
"isNsfw": false,
"categories": [],
"url": "https://www.venly.io/",
"imageUrl": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
},
"attributes": [
{
"type": "property",
"name": "Token Type ID",
"value": "2"
},
{
"type": "property",
"name": "Mint Number",
"value": "11"
}
],
"balance": 1,
"finalBalance": 1,
"transferFees": false
},
{
"id": "42",
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"imageUrl": "https://cdn.simplehash.com/assets/d5364a69e220124da8d406d3f49ee5c7ffbfb2b6ca84d73e3e99878dc28c0b8d.jpg",
"imagePreviewUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA",
"imageThumbnailUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA=s250",
"animationUrls": [],
"fungible": false,
"contract": {
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"symbol": "VENFCO",
"media": [
{
"type": "image",
"value": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
}
],
"type": "ERC_1155",
"verified": false,
"premium": false,
"isNsfw": false,
"categories": [],
"url": "https://www.venly.io/",
"imageUrl": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
},
"attributes": [
{
"type": "property",
"name": "Token Type ID",
"value": "2"
},
{
"type": "property",
"name": "Mint Number",
"value": "10"
}
],
"balance": 1,
"finalBalance": 1,
"transferFees": false
},
{
"id": "41",
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"imageUrl": "https://cdn.simplehash.com/assets/d5364a69e220124da8d406d3f49ee5c7ffbfb2b6ca84d73e3e99878dc28c0b8d.jpg",
"imagePreviewUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA",
"imageThumbnailUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA=s250",
"animationUrls": [],
"fungible": false,
"contract": {
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"symbol": "VENFCO",
"media": [
{
"type": "image",
"value": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
}
],
"type": "ERC_1155",
"verified": false,
"premium": false,
"isNsfw": false,
"categories": [],
"url": "https://www.venly.io/",
"imageUrl": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
},
"attributes": [
{
"type": "property",
"name": "Token Type ID",
"value": "2"
},
{
"type": "property",
"name": "Mint Number",
"value": "9"
}
],
"balance": 1,
"finalBalance": 1,
"transferFees": false
},
{
"id": "40",
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"imageUrl": "https://cdn.simplehash.com/assets/d5364a69e220124da8d406d3f49ee5c7ffbfb2b6ca84d73e3e99878dc28c0b8d.jpg",
"imagePreviewUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA",
"imageThumbnailUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA=s250",
"animationUrls": [],
"fungible": false,
"contract": {
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"symbol": "VENFCO",
"media": [
{
"type": "image",
"value": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
}
],
"type": "ERC_1155",
"verified": false,
"premium": false,
"isNsfw": false,
"categories": [],
"url": "https://www.venly.io/",
"imageUrl": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
},
"attributes": [
{
"type": "property",
"name": "Token Type ID",
"value": "2"
},
{
"type": "property",
"name": "Mint Number",
"value": "8"
}
],
"balance": 1,
"finalBalance": 1,
"transferFees": false
},
{
"id": "39",
"name": "Zap Village",
"description": "Enter the Venly Zap Village!",
"imageUrl": "https://cdn.simplehash.com/assets/d5364a69e220124da8d406d3f49ee5c7ffbfb2b6ca84d73e3e99878dc28c0b8d.jpg",
"imagePreviewUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA",
"imageThumbnailUrl": "https://lh3.googleusercontent.com/fz0nKPs5UOdsUmKYd2gotIkK-5N5ERyyDq8jgnC22dH1Pl-QfWMGEgL3mM8WLB7yQ_qy-REcZ3Z7q4RgR3PbuQbMRdIxS1NbDA=s250",
"animationUrls": [],
"fungible": false,
"contract": {
"name": "Venly NFT Collection",
"description": "This collection contains Venly NFTs.",
"address": "0xf0b30ec3720e9be1452b64d2ee476f57c2f535d3",
"symbol": "VENFCO",
"media": [
{
"type": "image",
"value": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
}
],
"type": "ERC_1155",
"verified": false,
"premium": false,
"isNsfw": false,
"categories": [],
"url": "https://www.venly.io/",
"imageUrl": "https://lh3.googleusercontent.com/UXW2okkylVnV1riV_L-4iR3S3Wwkjh1bgr5VhT7ZmK5ltai2HczB2cAAP45c5U89EcFwOgfH1ZMj69_NkQDeYZ4Fvy11hIbInbOJ"
},
"attributes": [
{
"type": "property",
"name": "Token Type ID",
"value": "2"
},
{
"type": "property",
"name": "Mint Number",
"value": "7"
}
],
"balance": 1,
"finalBalance": 1,
"transferFees": false
}
]
}
With only eleven API calls, you were able to create a user, create a wallet, and also mint NFTs to that wallet!
Preview NFTs on Sandbox/Testnet
Learn how to preview your NFTs on sandbox/testnet.

Schedule a demo with our team to explore tailored solutions or dive in and start building right away on our portal.
Updated 4 months ago